Here I share a simple example for exporting data in ASCII ‘csv’ file format. The file is saved to the folder that is set up in the web browser.
public static void main(Args _args)
{
Filename fileName = 'Filename.Txt';
AsciiStreamIo asciiIo = AsciiStreamIo::constructForWrite();
asciiIo.outRecordDelimiter("\r\n");
asciiIo.outFieldDelimiter(";");
asciiIo.write('Line 1');
asciiIo.write('Line 2');
File::SendFileToUser(asciiIo.getStream(),'asciiFile.txt');
}
Here is another example code. This one is reading / importing data in ASCII ‘csv’ file format. The csv file can be selected by the user from his or her local disk.
public static void main(Args _args)
{
FileUploadTemporaryStorageResult uploadFileResult = File::GetFileFromUser();
System.IO.Stream readStream = File::UseFileFromURL(uploadFileResult.getDownloadUrl());
AsciiStreamIo inFile = AsciiStreamIo::constructForRead(readStream);
container readCon;
str recordRead;
int nRead;
inFile.inFieldDelimiter("\r\n");
inFile.inRecordDelimiter("\r\n");
if (!inFile)
{
//FileName = uploadFileResult.getFileName();
Error('Cannot open file. Import failed.');
}
else
{
readCon = inFile.read();
while (inFile.status() == IO_Status::OK)
{
recordRead = conPeek(readCon, 1);
info(recordRead);
readCon = inFile.read();
nRead++;
}
}
}
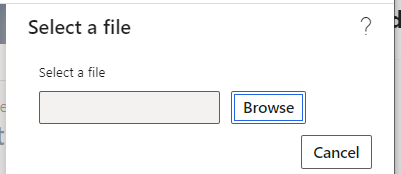
You can paste these codes to a runnable class and it will work or copy from https://github.com/PeterProkopecz/AX/tree/master/D365FO_FileReadWrite. You can customize them to fit your needs. Use ‘TextStreamIo’ class instead of ‘AsciiStreamIo’ in case you want to use normal text files.